Creating Disconnected Application in Xamarin.Forms using Monkey Cache 🐒
by Cedric Gabrang • Apr 15th 2020
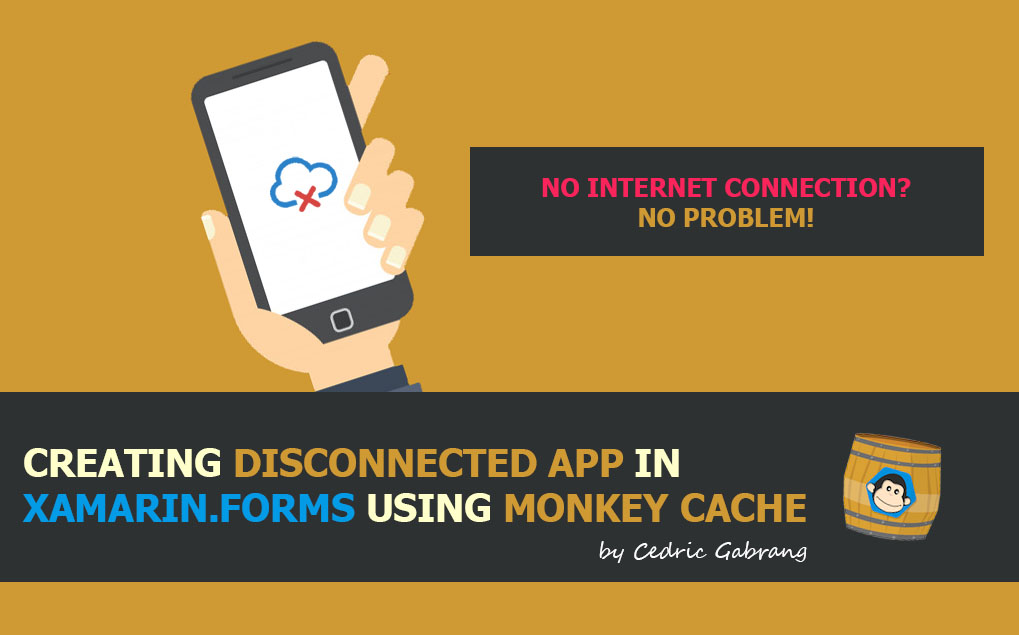
Every application needs to store data and almost every app developer running on the same
problem which is you have some data that you pulled from the internet or the user
entered it to the application, you need to save that data.
If you are looking to accomplish to make a web request, save the
result locally, and
have it expire after a given amount of time, that is basically what we
called
caching.
There are a lot of great solutions out there to cache data, but if you are looking for a
minimal amount of dependencies, you're on the right page,
mate!
In this article, I’m going to show you how to do data caching by using the Monkey Cache library by James
Montemagno.
What is Monkey Cache?
Monkey Cache enables you to easily store any type of data or just a simple string that you can easily serialize and deserialize back and forth. The key here is that you can set an expiration data associated with that data. So you can say this data should be used for the next few days.
Installing Monkey Cache
Monkey Cache consists of one core NuGet package (MonkeyCache) with three backing store providers. You can choose the backend that best fits your needs.
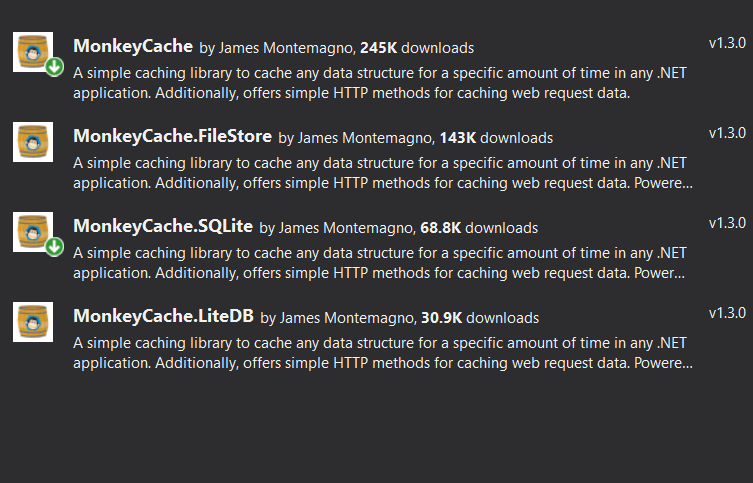
If you are already using one of these databases depending in your application, you may already have SQLite or LiteDB installed so these would be your natural choice.
Setup
Once Monkey Cache is installed in your project, it is time
to start storing objects.
Before calling any method, it is required that you set an ApplicationId
for
your
application so a folder is created specifically for your app on disk. This can be done
with a static string:
Barrel.ApplicationId = "your_unique_name_here";
Caching a Web Request
Let's have a web request and we get some
json
response from the server. What we want is
the ability to cache this
data incase the app
goes offline, but also we need it to expire after 24 hours.
static readonly HttpClient httpClient = new HttpClient(); public async Task> GetRandomJokesAsync() { var endpoint = string.Format("https://official-joke-api.appspot.com/jokes/programming/ten"); if(Connectivity.NetworkAccess == NetworkAccess.Internet) { HttpResponseMessage httpResponse = await httpClient.GetAsync(endpoint); string httpResult = httpResponse.Content.ReadAsStringAsync().Result; var httpData = JsonConvert.DeserializeObject >(httpResult); Barrel.Current.Empty(endpoint); Barrel.Current.Add(key: endpoint, data: httpData, TimeSpan.FromDays(1)); return httpData; } else { return Barrel.Current.Get >(endpoint); } }
The Add
method (which
also updates the item) takes in a
unique key, some data, and a time span
Demo
WITHOUT Monkey Cache - as you can see right here, the app is prone to crashing and seems like an empty screen except a message "Network error. Please check your connection".
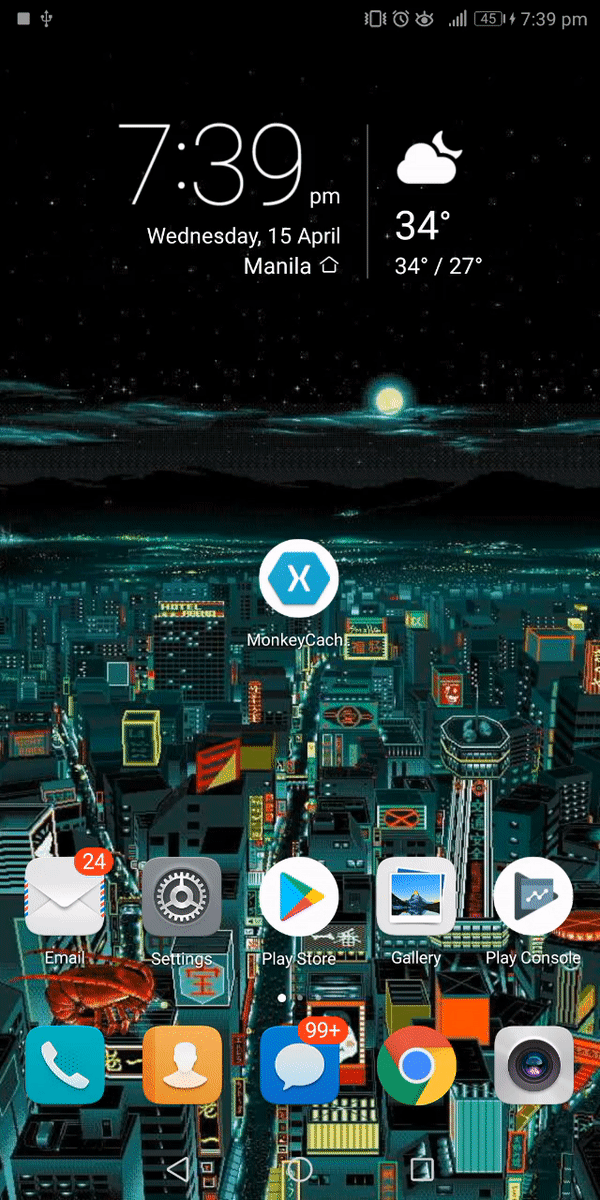
WITH Monkey Cache - Won’t it be a better user experience if you could see the latest data cached?
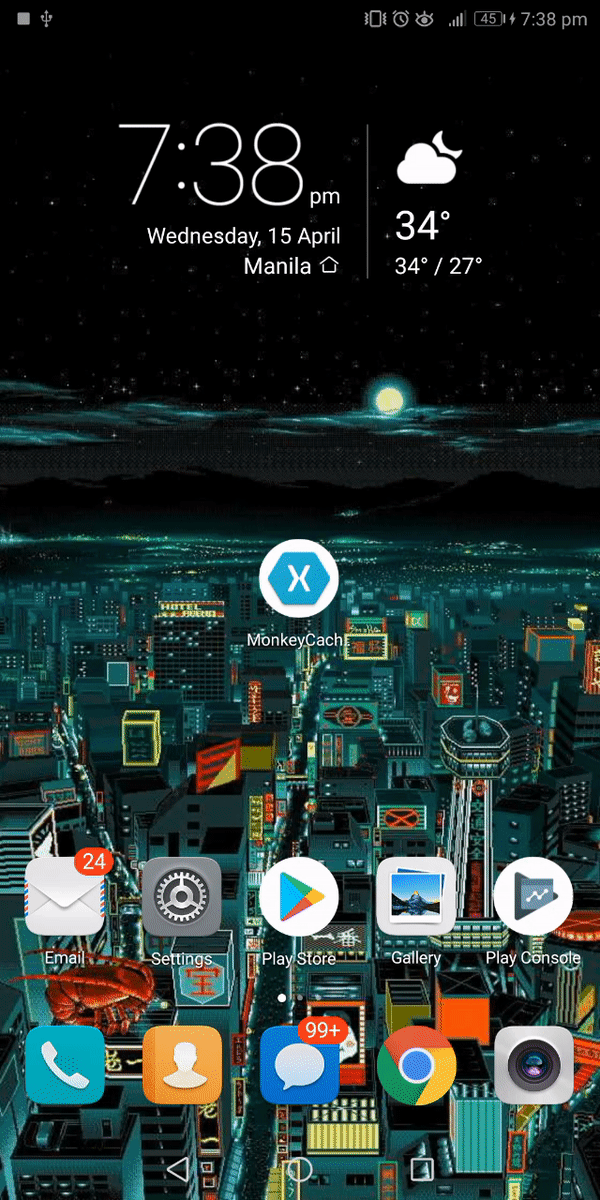